JavaScript is a versatile and powerful programming language that has become a cornerstone of modern web development. Whether you’re browsing a website, using a web application, or interacting with dynamic content online, JavaScript is likely at work behind the scenes. This beginner’s guide will introduce you to the basics of JavaScript, its key features, and how it is used to create interactive and dynamic web experiences.
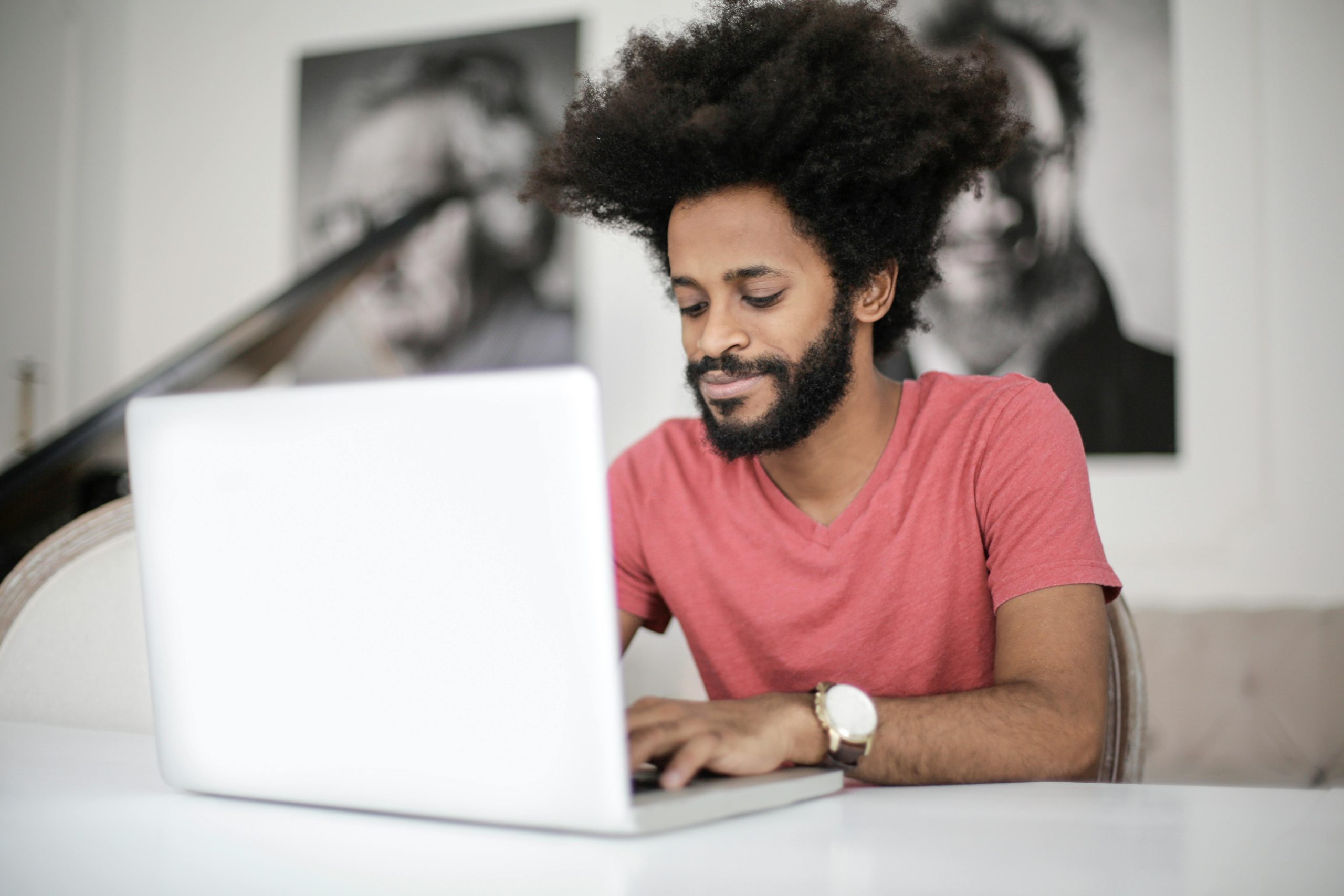
What is JavaScript?
JavaScript (often abbreviated as JS) is a high-level, interpreted programming language that conforms to the ECMAScript specification. It was created by Brendan Eich in 1995 while working at Netscape Communications. Initially designed to enhance web pages with dynamic features, JavaScript has evolved into a multi-paradigm language supporting object-oriented, imperative, and functional programming styles.
JavaScript is primarily known for its role in web development, but it is also used in other contexts, such as server-side programming (Node.js), mobile app development (React Native), and even in desktop applications (Electron).
Key Features of JavaScript
- Client-Side Scripting:
- JavaScript is predominantly used for client-side scripting, meaning it runs in the user’s web browser. This allows for creating interactive web pages that respond to user actions, such as clicks, form submissions, and hover effects.
- Dynamic Typing:
- JavaScript is a dynamically typed language, which means variables do not require a predefined data type. A variable can hold any data type and can change its type during execution.
- Prototype-Based Inheritance:
- Unlike classical object-oriented languages, JavaScript uses prototype-based inheritance. Objects can inherit properties and methods directly from other objects, allowing for more flexible and efficient inheritance patterns.
- First-Class Functions:
- In JavaScript, functions are first-class citizens. They can be assigned to variables, passed as arguments to other functions, and returned from functions. This feature is fundamental for functional programming and higher-order functions in JavaScript.
- Event-Driven Programming:
- JavaScript excels in handling events, making it ideal for creating responsive user interfaces. Event-driven programming allows developers to write code that reacts to various user interactions, such as clicks, keypresses, and mouse movements.
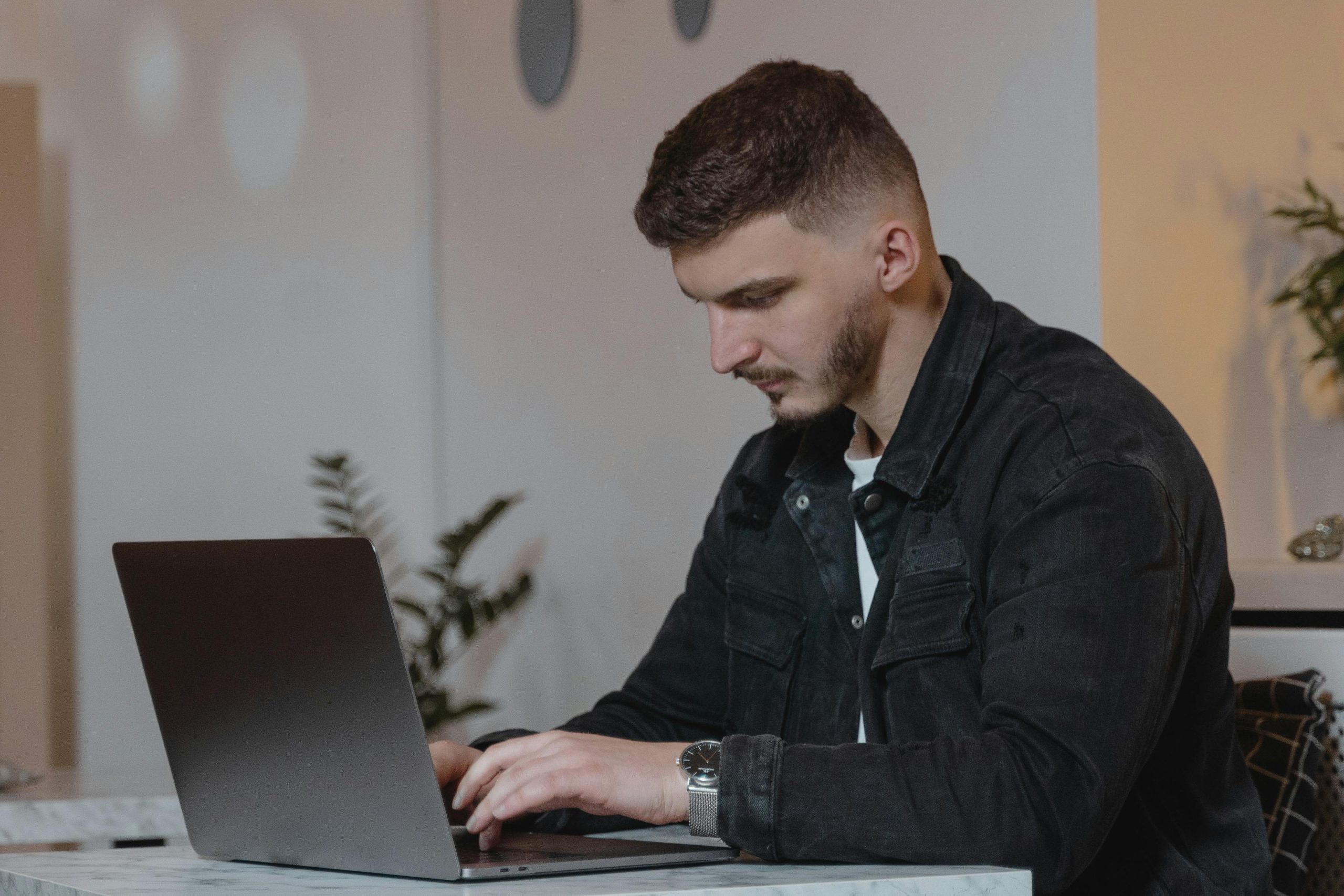
Basics of JavaScript Syntax
To start coding in JavaScript, you need to understand its basic syntax. Here are some fundamental concepts:
- Variables:
- Variables are used to store data. In JavaScript, you can declare variables using
var
,let
, orconst
.
var name = "John";
let age = 30;
const isStudent = true;
- Data Types:
- JavaScript supports several data types, including strings, numbers, booleans, objects, arrays, and more.
let message = "Hello, World!"; // String
let score = 95; // Number
let isActive = false; // Boolean
let user = { name: "Alice", age: 25 }; // Object
let colors = ["red", "green", "blue"]; // Array
- Functions:
- Functions are reusable blocks of code that perform specific tasks.
function greet(name) {
return "Hello, " + name;
}
console.log(greet("Alice")); // Output: Hello, Alice
- Control Structures:
- JavaScript provides control structures like if-else statements, loops, and switch statements to control the flow of the program.
let number = 10;
if (number > 0) {
console.log("Positive");
} else {
console.log("Negative");
}
for (let i = 0; i < 5; i++) {
console.log(i);
}
- DOM Manipulation:
- JavaScript allows you to manipulate the Document Object Model (DOM) to dynamically change the content and structure of web pages.
document.getElementById("myElement").innerHTML = "New Content";
JavaScript in Action
To see JavaScript in action, consider a simple example that changes the text of an HTML element when a button is clicked:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Example</title>
</head>
<body>
<h1 id="header">Welcome to JavaScript!</h1>
<button onclick="changeText()">Click Me</button>
<script>
function changeText() {
document.getElementById("header").innerHTML = "You clicked the button!";
}
</script>
</body>
</html>
In this example, when the button is clicked, the changeText
function is called, which changes the text of the h1
element.
Learning Resources
To further your understanding of JavaScript, consider exploring the following resources:
- Mozilla Developer Network (MDN): Comprehensive documentation and tutorials on JavaScript.
- W3Schools: Beginner-friendly tutorials and examples.
- FreeCodeCamp: Interactive coding challenges and projects.
- Codecademy: Interactive courses on JavaScript and web development.
JavaScript is an essential tool for modern web development, enabling the creation of dynamic and interactive web experiences. By mastering the basics of JavaScript, including variables, data types, functions, and DOM manipulation, you can begin building your own web applications. With numerous resources available, there’s no better time to start learning JavaScript and exploring its vast potential.