Scraping data from Facebook can be incredibly useful for research, sentiment analysis, or gaining business insights. However, due to Facebook’s strict policies and data security measures, scraping the platform requires careful consideration and the right tools. In this guide, we’ll explore how you can use Python to extract data from Facebook in a legal and responsible manner.
Understanding the Legal Aspects
Before starting, it’s important to understand Facebook’s terms of service. Scraping without permission may violate their policies, which could lead to account suspension. If you need data for business or research purposes, consider using Facebook’s official Graph API. If API access is not suitable, scraping methods should be used responsibly, ensuring that data retrieval remains ethical and legal.
Tools You’ll Need
To scrape Facebook using Python, you can use the following libraries:
- requests: For sending HTTP requests.
- BeautifulSoup: For parsing HTML content.
- Selenium: For automated browser interaction if necessary.
- facebook-sdk: If using the official Facebook API.
Method 1: Using the Facebook Graph API
For developers who need access to Facebook data, the Graph API is the most reliable method. Here’s how you can retrieve basic information using Python:
import requests
ACCESS_TOKEN = "your_access_token_here"
url = f"https://graph.facebook.com/v12.0/me?fields=id,name,email&access_token={ACCESS_TOKEN}"
response = requests.get(url)
data = response.json()
print(data) # Displays user information
To use the API, you need to create a Facebook Developer account and generate an access token. The API is subject to rate limits and permissions, so ensure your application has appropriate access rights.
Method 2: Scraping Facebook Using Selenium
If the data you need isn’t available through the API, Selenium can be used to automate browser actions. Keep in mind that scraping logged-in content might violate Facebook’s policies.
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import time
driver = webdriver.Chrome(executable_path="path_to_chromedriver")
driver.get("https://www.facebook.com/")
username = driver.find_element("name", "email")
password = driver.find_element("name", "pass")
username.send_keys("your_email@example.com")
password.send_keys("your_password")
password.send_keys(Keys.RETURN)
time.sleep(5) # Allow time for login
# Navigate and extract data
driver.quit()
Selenium opens a browser window, logs in to Facebook, and allows interaction with the website. You can then extract page content using BeautifulSoup or other parsing tools.
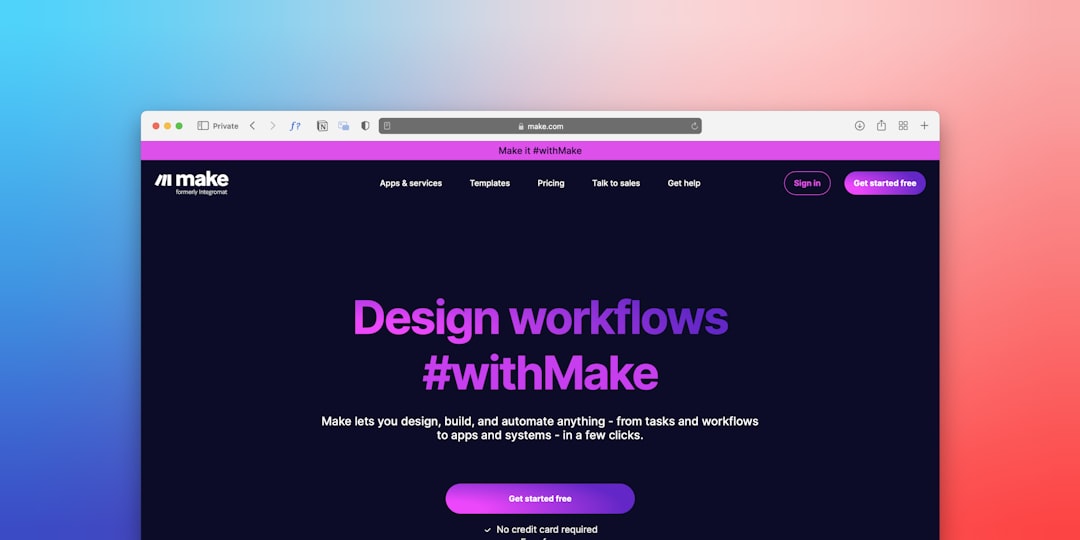
Alternative Methods: Using Public Data
Many Facebook pages and groups allow public access to their content. You can scrape this data without logging in. Here’s how you can fetch posts from a public page:
from bs4 import BeautifulSoup
import requests
page_url = "https://www.facebook.com/public_page_name"
headers = {"User-Agent": "Mozilla/5.0"}
response = requests.get(page_url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
for post in soup.find_all("div", class_="post_class"):
print(post.get_text())
Since Facebook frequently updates its HTML structure, you may need to adjust this code to match the latest webpage format.
Handling Anti-Scraping Measures
Facebook employs various mechanisms to prevent automated scraping, such as:
- Requiring login for content access.
- Rate limiting and CAPTCHA challenges.
- Dynamic content loading via JavaScript.
To deal with these challenges, you can:
- Use Selenium with headless mode to mimic a human browser.
- Rotate user agents and IPs to avoid detection.
- Respect Facebook’s robots.txt file and avoid excessive requests.
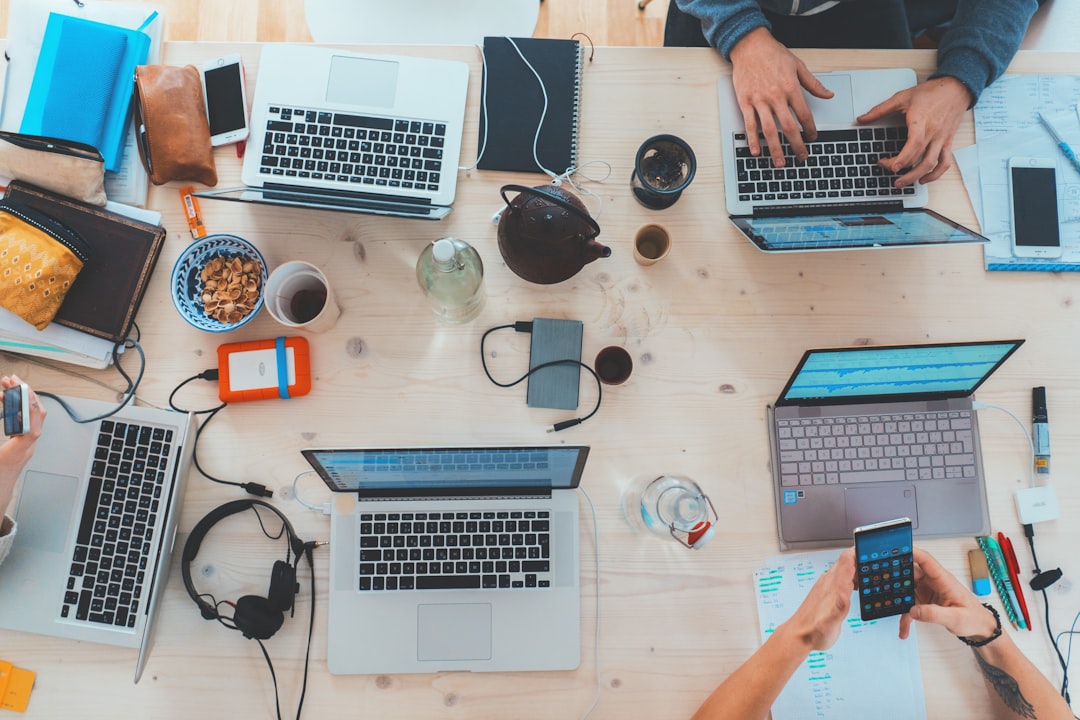
Best Practices
When scraping Facebook or any website, it’s crucial to follow ethical guidelines:
- Avoid scraping private data—Extract only publicly available information.
- Respect rate limits—Avoid overwhelming Facebook’s servers.
- Give credit—If using data for analysis or reports, cite the source.
Conclusion
Scraping Facebook using Python can be a powerful tool, but it’s essential to do it responsibly. The Facebook Graph API should be your first choice whenever possible. If you need to scrape content manually, tools like Selenium and BeautifulSoup can help, but you must navigate Facebook’s anti-scraping measures carefully. Always respect terms of service and ethical guidelines to avoid potential legal issues.
Are you planning to scrape Facebook for research or business? Consider trying the official API first, and if that doesn’t suit your needs, use automation responsibly to stay within ethical boundaries.