When working with React, developers sometimes encounter the error message: “Objects are not valid as a React child.” This error can be confusing, especially for beginners. It usually occurs when rendering an object instead of valid children like strings, numbers, or React elements. Understanding the causes and solutions will help in debugging React applications efficiently.
Understanding the Error
React components can only render valid child elements, such as:
- Strings
- Numbers
- React components
- Arrays containing valid React children
However, when trying to render an object directly, React does not know how to display it, which leads to this error.
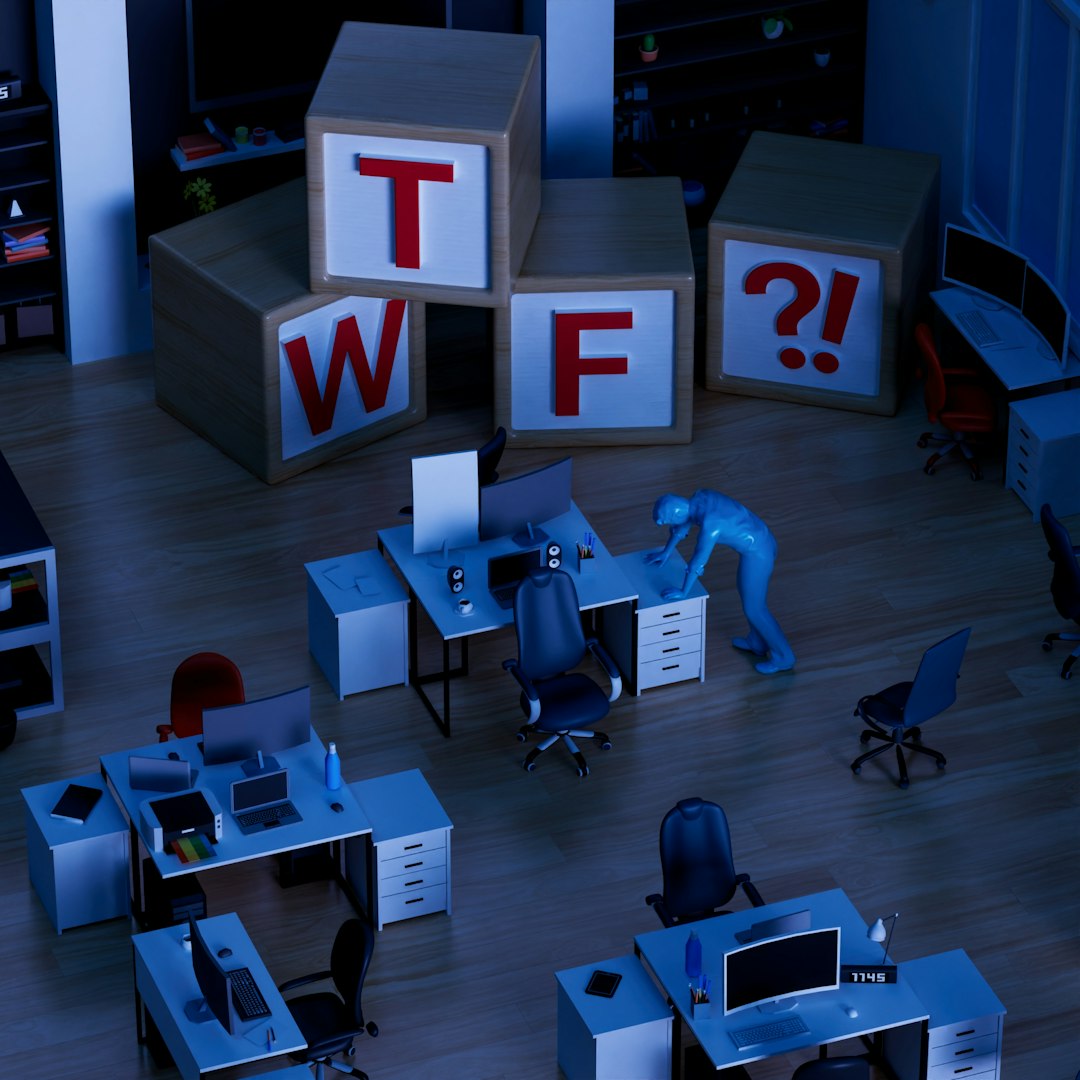
Common Causes
Several common mistakes can trigger this error:
1. Attempting to Render an Object
A frequent mistake is trying to output an object directly inside JSX:
const user = { name: "John", age: 30 }; return <p>{user}</p>; // ❌ This will cause an error
React does not know how to convert an object into a renderable format.
2. Accessing Undefined or Null Values
If a variable is expected to hold a string or component but unintentionally holds an object, this error can occur:
const data = null; return <div>{data.message}</div>; // ❌ data is null, leading to an error
3. Incorrect API Data Handling
When fetching data from an API, it’s crucial to ensure the response is correctly structured before rendering:
fetch('https://api.example.com/user') .then(response => response.json()) // Ensure JSON parsing .then(data => setUser(data)); return <p>{user}</p>; // ❌ Directly rendering an object will cause an error
How to Fix the Error
There are several ways to fix this issue:
1. Convert Objects into Strings
If displaying object content is necessary, convert it into a string using JSON.stringify
:
const user = { name: "Jane", age: 25 }; return <p>{JSON.stringify(user)}</p>; // ✅ Displays object content as a string
2. Access Object Properties
Instead of trying to render an entire object, extract and render individual properties:
const user = { name: "Jane", age: 25 }; return <p>{user.name}, {user.age}</p>; // ✅ Correct approach
3. Ensure Proper API Handling
When rendering data from an API request, always check for undefined or incorrect values:
return <p>{user ? user.name : "Loading..." }</p>; // ✅ Handles possible null/undefined values
4. Debug Data Structures
If unsure about what is being rendered, logging the data before rendering can be helpful:
console.log(user);
This helps identify whether an object is unintentionally being passed to JSX.
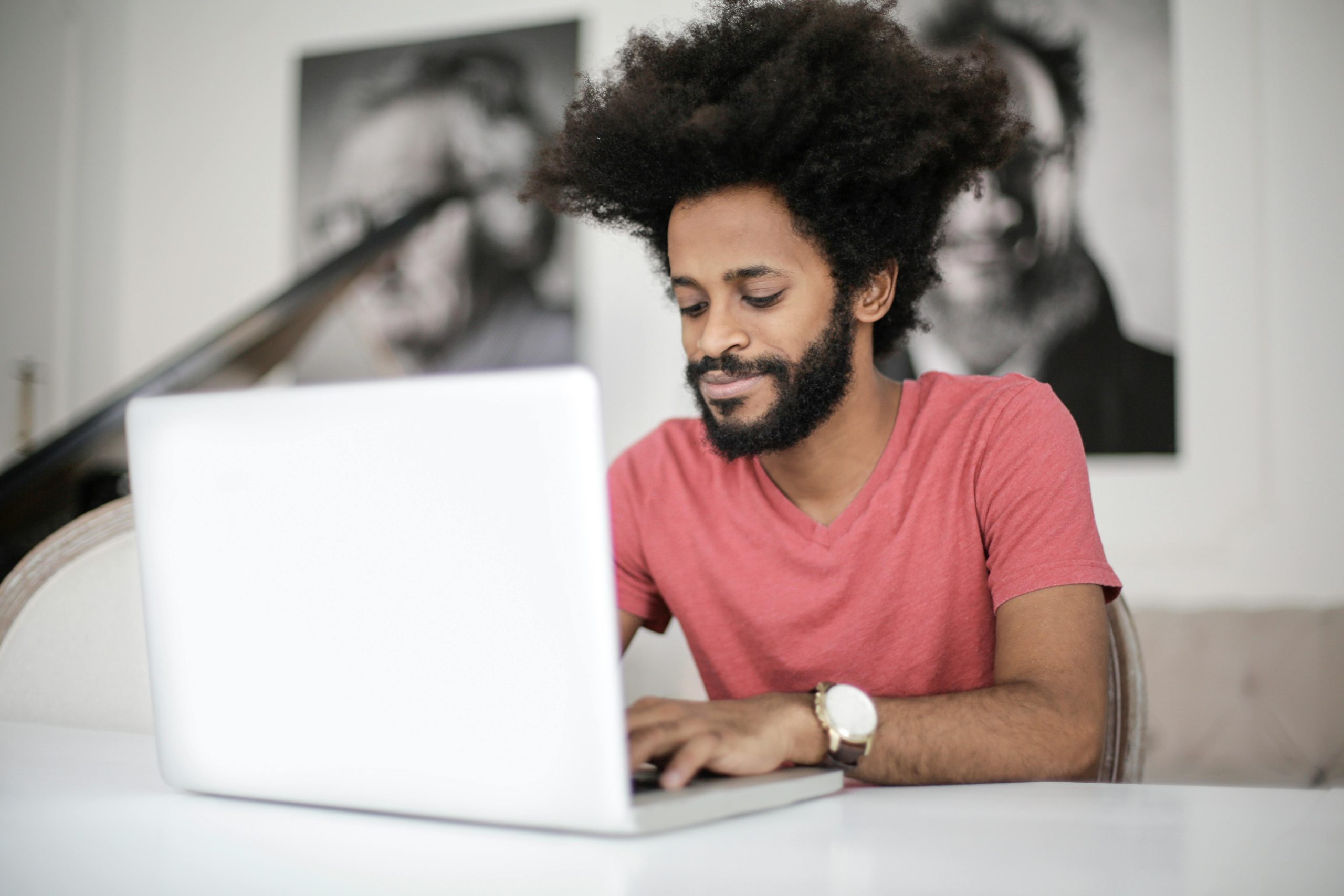
Frequently Asked Questions
1. Why does this error occur in React?
React expects children to be strings, numbers, or valid elements. Objects are not directly renderable, leading to this error.
2. Can arrays be rendered in JSX?
Yes, but the array must contain valid React children. Objects inside an array will also trigger the error.
3. How do I check what is causing the error?
Using console.log
before returning a JSX element can help identify whether an object is being unexpectedly passed.
4. What should I do if I get this error from an API response?
Parse API responses correctly and ensure that you are rendering individual properties rather than entire objects.
5. Is JSON.stringify a good long-term solution?
It can be useful for debugging but should not be a permanent solution in production-ready applications.
By understanding this error and its solutions, React developers can ensure smoother development experiences and avoid unnecessary debugging time.